Posted by: Shalu T D
This article discusses some of the standard library changes that come under the Kotlin 1.5 version. Please find each of them below.
1. New collection functions
Consider you have a list of objects, all with a nullable property, and you want to get the first non-null value of that property in the collection. Usually, you would first use mapNotNull() to get a collection of all non-null values and then call
firstOrNull() to get the final value.
In Kotlin 1.5 we have functions firstNotNullOf()and
firstNotNullOfOrNull(). The function
firstNotNullOf() returns first non-null value of the passed selector, or exception thrown if no element matches. The function
firstNotNullOfOrNull() returns the first non-null value of the passed selector, or null if no element matches.
The functionfirstNotNullOf() is the combination of
mapNotNull() and
first(). The same way
firstNotNullOfOrNull() is the combination of
mapNotNull() and
firstOrNull().
Please check the below code for more information.
data class Employee(val name: String, val designation: String?)val employees = listOf( Employee("Felix", null), Employee("Vee", null), Employee("Ram", "Developer"), Employee("Vivek", "Senior Developer"), Employee("Joe", "Developer"), )// Old method val firstDesignation = employees.mapNotNull { it.designation }.firstOrNull()// Kotlin 1.5 method val newfirstDesignation = employees.firstNotNullOfOrNull { it.designation }
2. Unsigned integer types Ubyte, UShort, UInt and ULong
These types of unsigned numbers are useful wherever you want to constrain a type to only contain non-negative values. They can also be more intuitive when you are working on bit and byte level, manipulating pixels in bitmaps, the bytes in a file, or other binary data.
UByte
: an unsigned 8-bit integer, ranges from 0 to 255UShort
: an unsigned 16-bit integer, ranges from 0 to 65535UInt
: an unsigned 32-bit integer, ranges from 0 to 2^32 – 1ULong
: an unsigned 64-bit integer, ranges from 0 to 2^64 – 1
3. Integer floor division and mod operator
The new function floorDiv() provides floored division on integers. The floored division always rounds the result down.
(5).floorDiv(3) -> returns 1 (-5).floorDiv(3) -> returns -2
The remainder function, called ‘mod’ returns the remainder of the floored division. This is entirely different from the ‘%’ operator, called ‘rem’ in Kotlin.
Consider the below code,
val a = -4 val b = 3println(-4 % 3) -> -1 println(a.mod(b)) -> 2
Here, the ‘%’ operation returns remainder as -1. But a.mod(b) is the difference between a and a.floorDiv(b) * b. It’s either zero or has the same sign as b, while a % b can have a different one.
4. char-to-integer conversion API
For individual characters, Kotlin introduced functions that make their conversion into numbers more expressive. When you are converting a character into a number, you can mean one of two things: you either mean the char code for the character in question, or you mean the actual digit the character represents, parsing the number. Both of these can be concisely express with the new conversion API. It introduced Char
conversions that are divided into the following sets of clearly named functions:
- Functions to get the integer code of
Char
and to constructChar
from the given code:
fun Char(code: Int): Char fun Char(code: UShort): Char val Char.code: Int
- Functions to convert
Char
to the numeric value of the digit it represents:
fun Char.digitToInt(radix: Int): Int fun Char.digitToIntOrNull(radix: Int): Int?
- An extension function for
Int
to convert the non-negative single digit it represents to the correspondingChar
representation:
fun Int.digitToChar(radix: Int): Char
Job Offers
5. Char category in multiplatform code
Provided a number of functions to check character properties available in common code. They help you when you want to check if a character is a digit, a letter, or either one of them, whether a letter is an upper, lower, or title case, and if its Unicode representation is defined or an ISO sequence.
'1'.isDigit() -> true 'A'.isLetter() -> true '!'.isLetterOrDigit() -> false 'C'.isLowerCase() -> false 'C'.isUpperCase() -> true 'b'.isTitleCase() -> false '?'.isDefined() -> true '\n'.isISOControl() -> true
String conversion functions:-
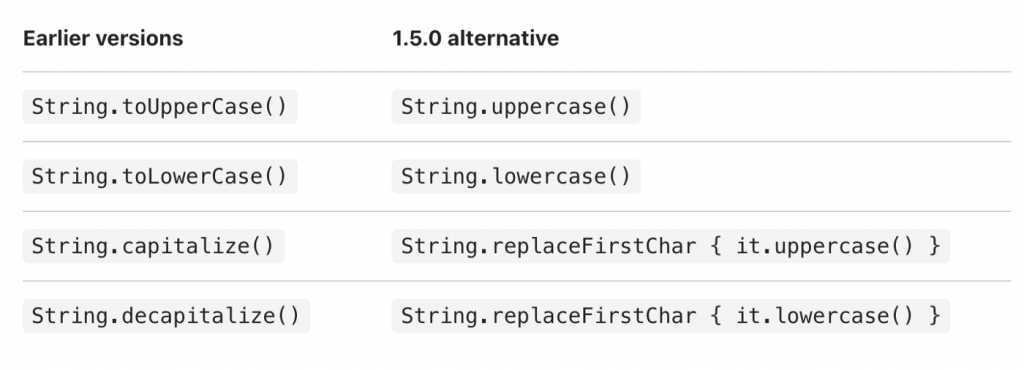
Char conversion functions:-

If you want locale-based conversion, you need to explicitly specify the locale as below.
“kotlin”.uppercase(Locale.forLanguageTag(“tr-TR”))
7. Strict version of String?.toBoolean()
Introduced String.toBooleanStrict()
and String.toBooleanStrictOrNull()
functions. The functionString.toBooleanStrict()
throws exception for all inputs other than that of ‘true’ or ‘false’ literals. This is case sensitive too. As it is case-sensitive, the example "true".toBooleanStrict()
gives output as true but "True".toBooleanStrict()
throws exceptions.
String.toBooleanStrictOrNull()
will not throw an exception, instead, it gives null
for all inputs other than that of ‘true’ or ‘false’ literals. This is also case-sensitive.
"true".toBooleanStrictOrNull()
-> returns true
"True".toBooleanStrictOrNull()
-> returns null
I hope you learned some of the Kotlin standard library changes here. If you need to know more about this, you can use the documentation.
If you need any help, join me on LinkedIn and Github. If you liked this article, share it with fellow Kotlin developers.
Now you are ready to go. Thanks for reading!